Overview
This project is a stock price prediction application built using LSTM (Long Short-Term Memory) neural networks to predict stock prices based on historical data. It uses Yahoo Finance API to fetch data and displays 7-day forecasts with intuitive graph visualization.
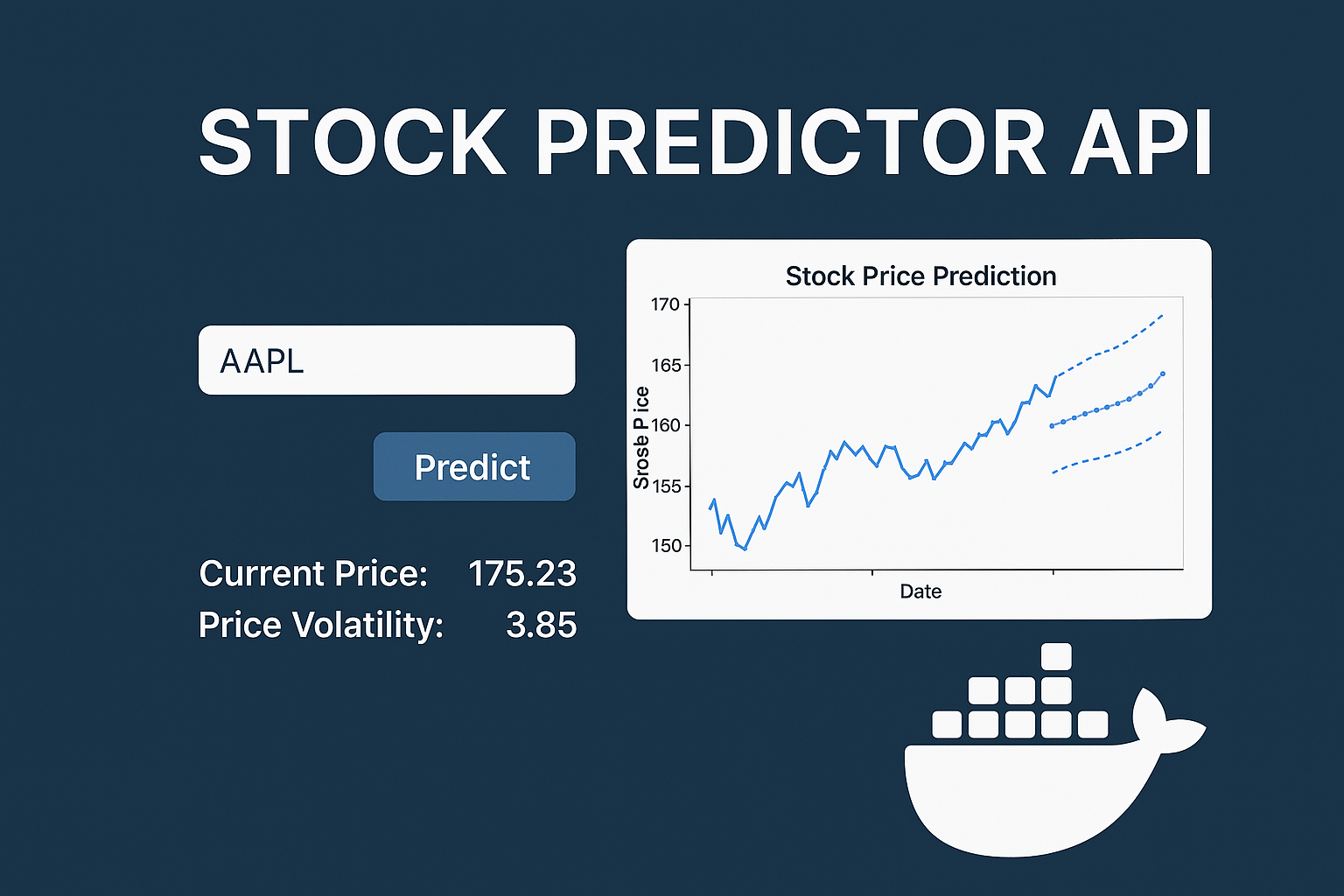
Project Structure
stock-predictor/
├── app/ # Flask app
│ ├── templates/
│ ├── static/
│ ├── main.py # Flask routes
│ └── model.py # LSTM logic
├── Dockerfile
├── docker-compose.yml
├── requirements.txt
└── README.md
Docker Setup
The application is containerized using Docker to ensure consistent development and deployment. After cloning the repository, use the following commands to build and run the container:
docker-compose build --no-cache
docker-compose up
Once running, the app is accessible via http://127.0.0.1:5050
.
Below is an example of the terminal output after successfully running the application:
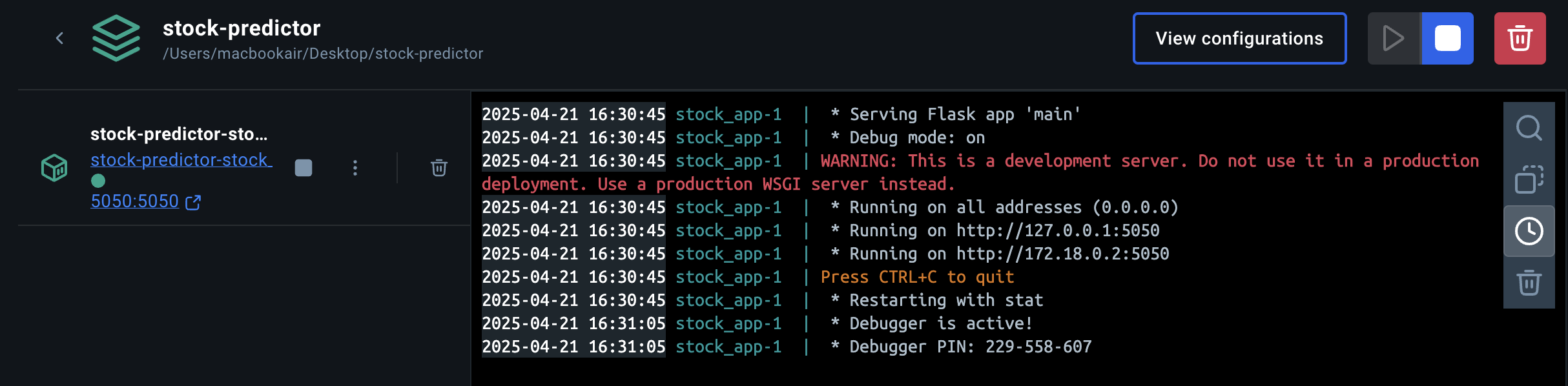
Flask API Structure
The core backend logic is built using Flask. It defines two main routes:
- GET / - Renders the homepage with a form to enter a stock ticker
- POST /predict - Receives the ticker symbol, triggers LSTM prediction, and returns results in JSON
The prediction results include:
predicted_prices
: List of predicted closing prices for the next 7 daysvolatility
: Difference between max and min predicted pricesgraph_url
: URL to generated graph imagecurrent_price
: Latest real-time stock price
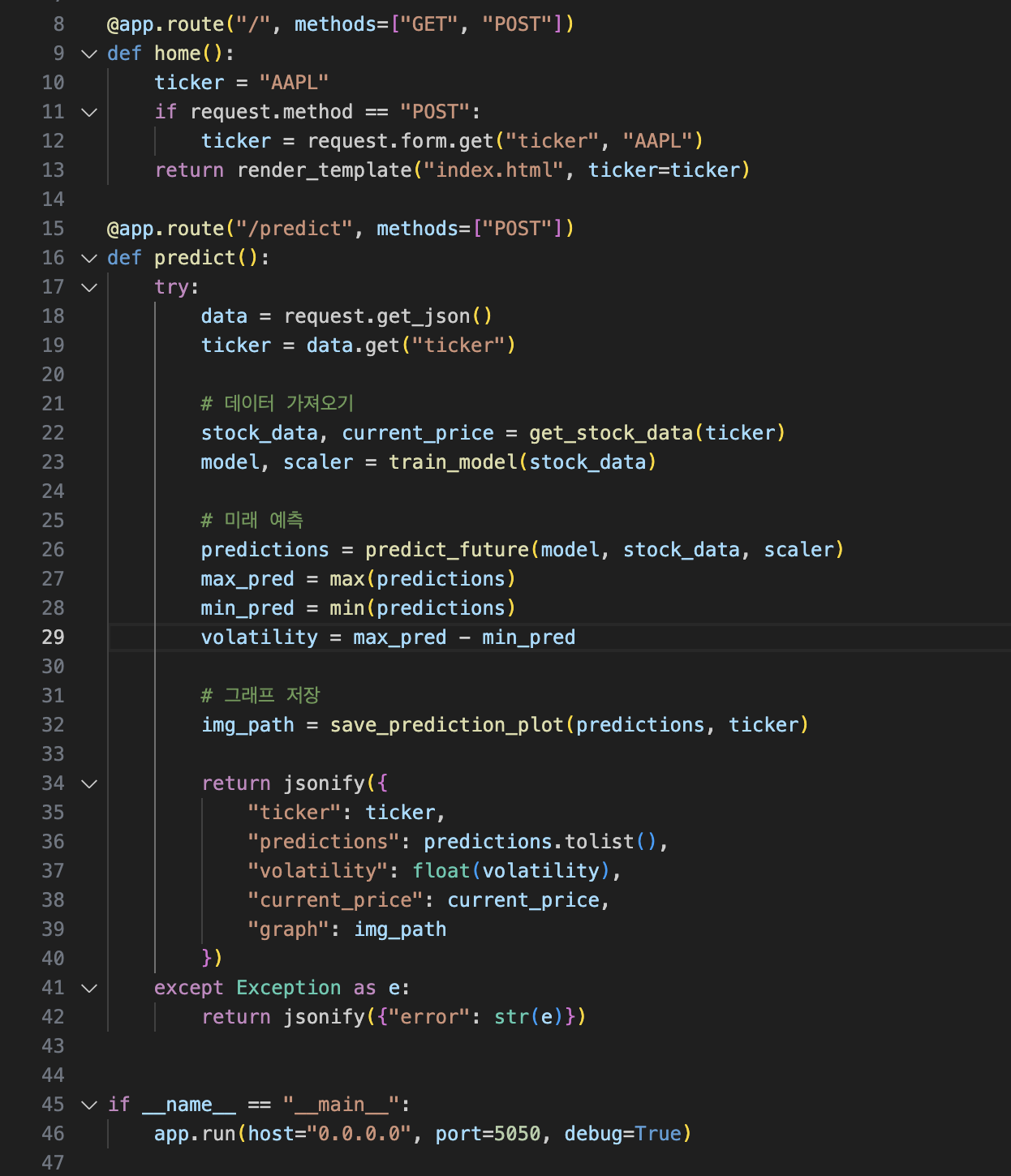
▲ main.py
showing Flask route logic for receiving and returning predictions.
LSTM Prediction Model
The LSTM model is implemented in model.py
using TensorFlow. It processes time-series stock price data and predicts the next 7 days.
- Data is normalized and reshaped into LSTM-compatible sequences
- The trained model outputs future price predictions
- The results are saved and plotted using Matplotlib
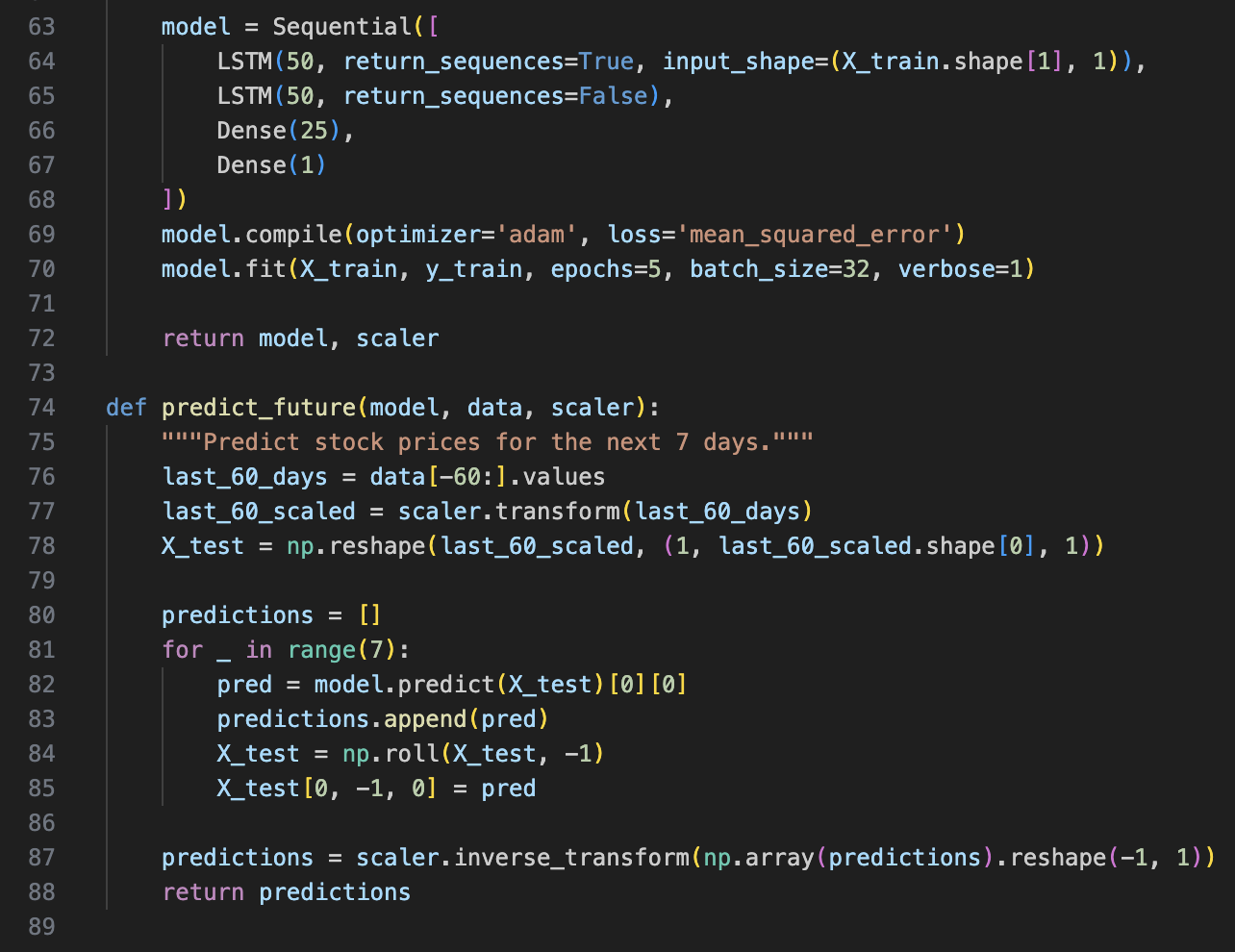
▲ Key portion of LSTM implementation and prediction logic in model.py
.
Key Features
- 7-Day Price Prediction: LSTM model forecasts upcoming prices
- Graph Visualization: Visual output using Matplotlib
- Volatility Measurement: Calculates and displays price fluctuation
- Current Price Display: Shows most recent market price
- Dockerized: Entire app runs inside a container
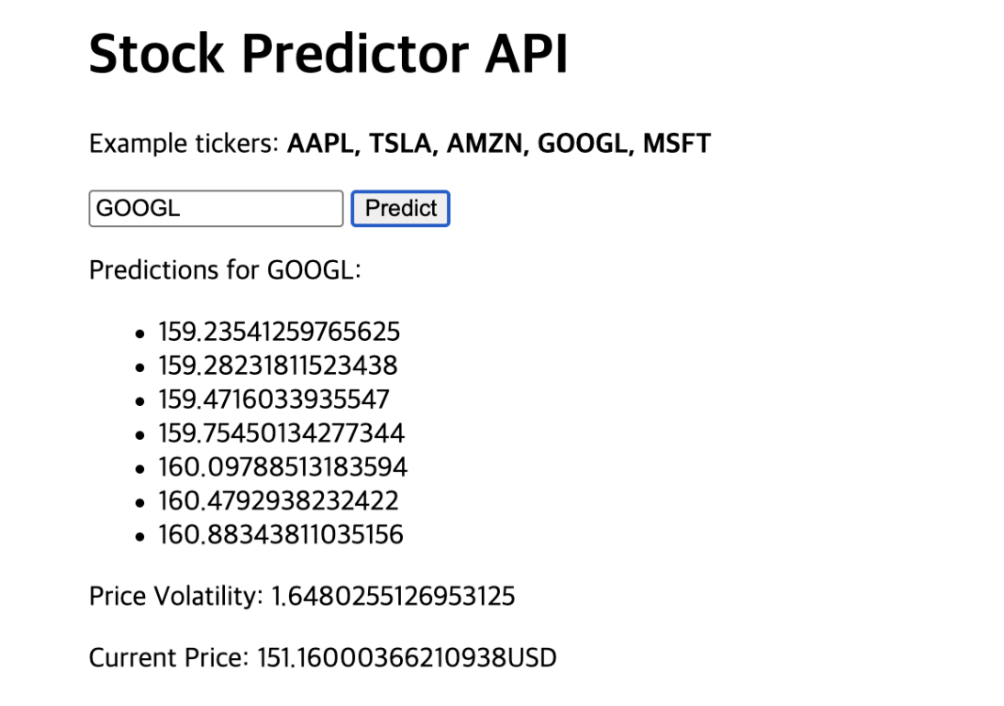
How It Works
The application fetches stock data using yfinance and trains a TensorFlow LSTM model. After prediction, it generates a graph and returns results via a Flask API.
- GET /: Homepage with input for ticker
- POST /predict: Returns JSON with prices, graph URL, current price, and volatility
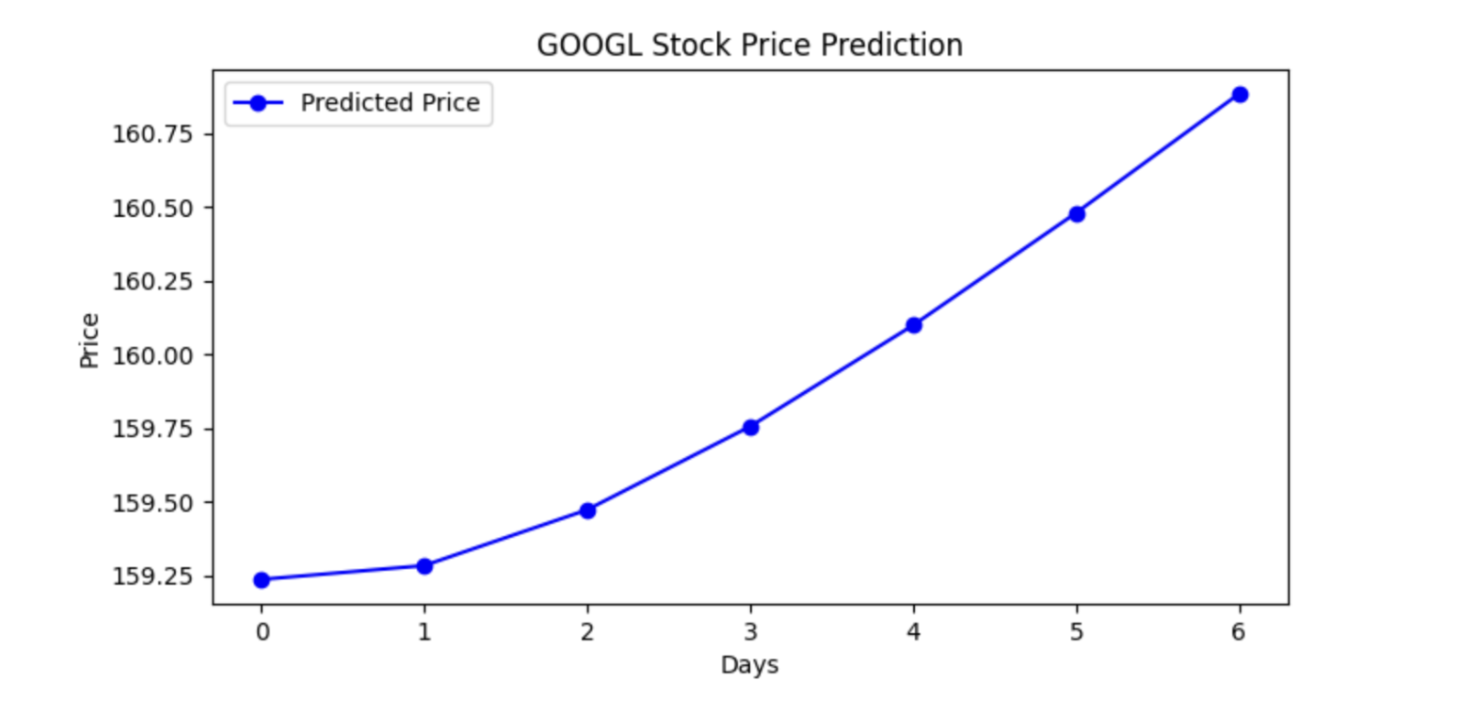
Running with Docker
- Clone the repo:
git clone https://github.com/pkisung/stock-predictor
- Build image:
docker-compose build --no-cache
- Run:
docker-compose up -d
- Visit:
http://127.0.0.1:5050/
Configuration Files
The following files configure how the environment is built and dependencies are managed:
Dockerfile
- Uses
python:3.9
as the base image - Copies project files and installs dependencies
- Exposes port
5050
and runsmain.py
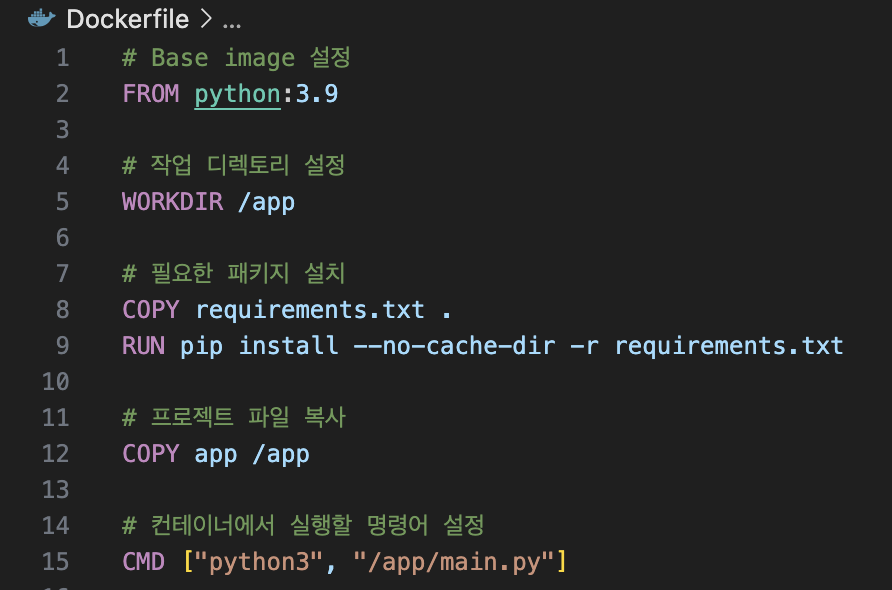
▲ Core Dockerfile commands used to containerize the app
docker-compose.yml
- Defines the service name and build context
- Maps port
5050
to local machine
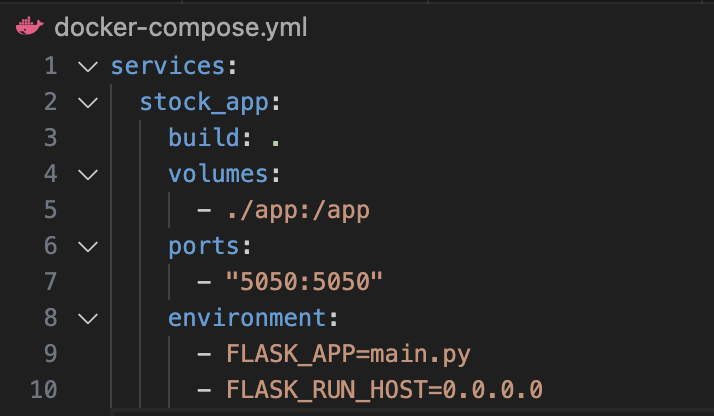
▲ Sample docker-compose configuration
requirements.txt
- Lists essential Python libraries such as Flask, TensorFlow, Matplotlib, and yfinance
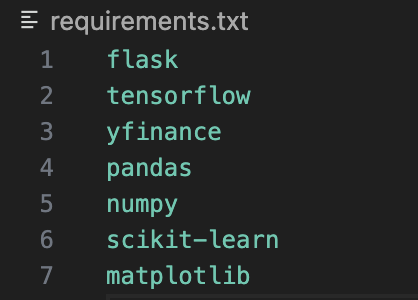
▲ Required packages for the application
More Details & Source Code
Explore the full codebase and documentation on GitHub: